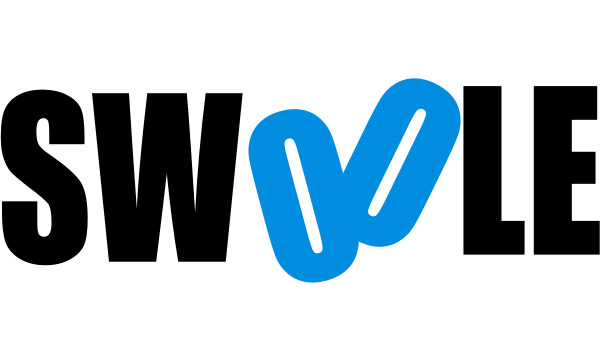
Swoole is a powerful extension for PHP that supports asynchronous I/O operations and coroutines. It is designed to significantly improve the performance of PHP applications by enabling the creation of high-performance, asynchronous, and parallel network applications. Swoole extends the capabilities of PHP beyond what is possible with traditional synchronous PHP scripts.
Key Features of Swoole
-
Asynchronous I/O:
- Swoole offers asynchronous I/O operations, allowing time-consuming I/O tasks (such as database queries, file operations, or network communication) to be performed in parallel and non-blocking. This leads to better utilization of system resources and improved application performance.
-
Coroutines:
- Swoole supports coroutines, allowing developers to write asynchronous programming in a synchronous style. Coroutines simplify the handling of asynchronous code, making it more readable and maintainable.
-
High Performance:
- By using asynchronous I/O operations and coroutines, Swoole achieves high performance and low latency, making it ideal for applications with high-performance demands, such as real-time systems, WebSockets, and microservices.
-
HTTP Server:
- Swoole can function as a standalone HTTP server, offering an alternative to traditional web servers like Apache or Nginx. This allows PHP to run directly as an HTTP server, optimizing application performance.
-
WebSockets:
- Swoole natively supports WebSockets, facilitating the creation of real-time applications like chat applications, online games, and other applications requiring bidirectional communication.
-
Task Worker:
- Swoole provides task worker functionality, enabling time-consuming tasks to be executed asynchronously in separate worker processes. This is useful for handling background jobs and processing large amounts of data.
-
Timer and Scheduler:
- With Swoole, recurring tasks and timers can be easily managed, allowing for efficient implementation of timed tasks.
Example Code for a Simple Swoole HTTP Server
<?php
use Swoole\Http\Server;
use Swoole\Http\Request;
use Swoole\Http\Response;
$server = new Server("0.0.0.0", 9501);
$server->on("start", function (Server $server) {
echo "Swoole HTTP server is started at http://127.0.0.1:9501\n";
});
$server->on("request", function (Request $request, Response $response) {
$response->header("Content-Type", "text/plain");
$response->end("Hello, Swoole!");
});
$server->start();
In this example:
- An HTTP server is started on port 9501.
- For each incoming request, the server responds with "Hello, Swoole!".
Benefits of Using Swoole
- Performance: Asynchronous I/O and coroutines allow applications to handle many more simultaneous connections and requests, significantly improving scalability and performance.
- Resource Efficiency: Swoole enables more efficient use of system resources compared to synchronous PHP scripts.
- Flexibility: With Swoole, developers can write complex network applications, real-time services, and microservices directly in PHP.
Use Cases for Swoole
- Real-Time Applications: Chat systems, notification services, online games.
- Microservices: Scalable and high-performance backend services.
- API Gateways: Asynchronous processing of API requests.
- WebSocket Servers: Bidirectional communication for real-time applications.
Swoole represents a significant extension of PHP's capabilities, enabling developers to create applications that go far beyond traditional PHP use cases.